๋น๋๊ธฐ๋ฅผ ๋ณ๋ ฌ์ ์ผ๋ก ์ํํ๋ ๊ฒ์๋ ํฌ๊ฒ 3๊ฐ์ง ๋ฐฉ๋ฒ์ด ์์ต๋๋ค.
1. Promise.all
์ํ ๊ฐ๋ฅํ ๊ฐ์ฒด์ ์ฃผ์ด์ง ๋ชจ๋ ํ๋ก๋ฏธ์ค๊ฐ ์ดํํ ํ, ํน์ ํ๋ก๋ฏธ์ค๊ฐ ์ฃผ์ด์ง์ง ์์์ ๋ ์ดํํ๋ Promise๋ฅผ ๋ฐํํฉ๋๋ค. ์ฃผ์ด์ง ํ๋ก๋ฏธ์ค ์ค ํ๋๊ฐ ๊ฑฐ๋ถํ๋ ๊ฒฝ์ฐ, ์ฒซ ๋ฒ์งธ๋ก ๊ฑฐ์ ํ ํ๋ก๋ฏธ์ค์ ์ด์ ๋ฅผ ์ฌ์ฉํด ์์ ๋ ๊ฑฐ๋ถํฉ๋๋ค.
์ ๋ ฅ ๊ฐ์ผ๋ก ๋ค์ด์จ ํ๋ก๋ฏธ์ค ์ค ํ๋๋ผ๋ ๊ฑฐ๋ถ ๋นํ๋ฉด Promise.all()์ ์ฆ์ ๊ฑฐ๋ถํฉ๋๋ค.
const promise1 = Promise.resolve(3);
const promise2 = 42;
const promise3 = new Promise((resolve, reject) => {
setTimeout(resolve, 100, 'foo');
});
Promise.all([promise1, promise2, promise3]).then((values) => {
console.log(values);
});
// Expected output: Array [3, 42, "foo"]
2. Promise.allSettled
Promise.allSettled() ๋ฉ์๋๋ ์ฃผ์ด์ง ๋ชจ๋ ํ๋ก๋ฏธ์ค๋ฅผ ์ดํํ๊ฑฐ๋ ๊ฑฐ๋ถํ ํ, ๊ฐ ํ๋ก๋ฏธ์ค์ ๋ํ ๊ฒฐ๊ณผ๋ฅผ ๋ํ๋ด๋ ๊ฐ์ฒด ๋ฐฐ์ด์ ๋ฐํํฉ๋๋ค.
์ผ๋ฐ์ ์ผ๋ก ์๋ก์ ์ฑ๊ณต ์ฌ๋ถ์ ๊ด๋ จ ์๋ ์ฌ๋ฌ ๋น๋๊ธฐ ์์ ์ ์ํํด์ผ ํ๊ฑฐ๋, ํญ์ ๊ฐ ํ๋ก๋ฏธ์ค์ ์คํ ๊ฒฐ๊ณผ๋ฅผ ์๊ณ ์ถ์ ๋ ์ฌ์ฉํฉ๋๋ค.
์๋ฅผ ๋ค์ด, ์์ฒญ๋๊ฒ ๋ง์ ์์ ๋ฐ์ดํฐ๋ฅผ ์ ๋ก๋ ํด์ผ ํ๊ณ , ๊ทธ ์ค ์ ๋ก๋์ ์คํจํ ํ์ผ๋ค๋ง ๋ชจ์์ ๋ค์ ์ ๋ก๋๋ฅผ ํ๊ณ ์ถ๋ค๋ฉด Promise.allSettled๋ฅผ ์ฌ์ฉํ ์ ์๋ค.
Promise.allSettled๋ฅผ ์ฌ์ฉํ๋ค๋ฉด
1. ๋น๋๊ธฐ ์์ฒญ์ ๋ณ๋ ฌ์ ์ผ๋ก ์ฒ๋ฆฌํด์ ์ ๋ก๋ ์๋๋ฅผ ๋จ์ถํ ์ ์๊ณ ,
2. resolve, reject ๋ชจ๋ ๋น๋๊ธฐ ์์ฒญ์ด ๋๋ ๋๊น์ง ๊ธฐ๋ค๋ฆฐ ํ์ ๊ฒฐ๊ณผ ๋ฐํํ๊ธฐ ๋๋ฌธ์ ์คํจํ ํ์ผ๋ค๋ง ์ฐพ์๋ผ ์ ์๋ค.
const promise1 = Promise.resolve(3);
const promise2 = new Promise((resolve, reject) =>
setTimeout(reject, 100, 'foo'),
);
const promises = [promise1, promise2];
Promise.allSettled(promises).then((results) =>
results.forEach((result) => console.log(result.status)),
);
// Expected output:
// "fulfilled"
// "rejected"
Promise.all์ ์ฌ์ฉํด์ ์ด๋ฐ์์ผ๋ก Promsie.allSettled๋ฅผ ๊ตฌํํ ์๋ ์๋ค.
function allSettled(promises) {
let wrappedPromises = promises.map(p => Promise.resolve(p)
.then(
val => ({ status: 'fulfilled', value: val }),
err => ({ status: 'rejected', reason: err })));
return Promise.all(wrappedPromises);
}
3. Promise.race
iterable ์์ ์๋ ํ๋ก๋ฏธ์ค ์ค์ ๊ฐ์ฅ ๋จผ์ ์๋ฃ๋ ๊ฒ์ ๊ฒฐ๊ณผ๊ฐ์ผ๋ก ๊ทธ๋๋ก ์ดํํ๊ฑฐ๋ ๊ฑฐ๋ถํฉ๋๋ค.
const promise1 = new Promise((resolve, reject) => {
setTimeout(resolve, 500, 'one');
});
const promise2 = new Promise((resolve, reject) => {
setTimeout(resolve, 100, 'two');
});
Promise.race([promise1, promise2]).then((value) => {
console.log(value);
// Both resolve, but promise2 is faster
});
// Expected output: "two"
์ ์ธ๊ฐ์ง ๋ฉ์๋ ๋ชจ๋ ๋๋ถ๋ถ์ ๋ธ๋ผ์ฐ์ ์์ ํธํ๋๋ค.
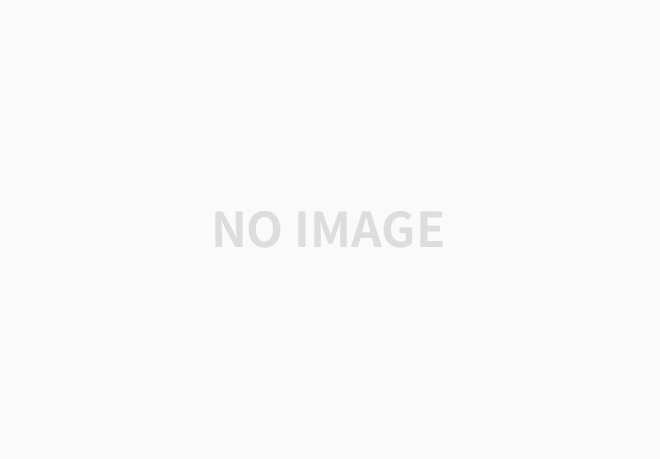
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise/all
https://stackoverflow.com/questions/30569182/promise-allsettled-in-babel-es6-implementation
'๐ป ํ๋ก ํธ์๋ > ๐ JS' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[์๋ฐ์คํฌ๋ฆฝํธ] ๋น๋๊ธฐ ์ฒ๋ฆฌ์ ์ด๋ฒคํธ๋ฃจํ (0) | 2023.12.27 |
---|---|
[์๋ฐ์คํฌ๋ฆฝํธ] ํด๋ก์ (1) | 2023.12.27 |
[์๋ฐ์คํฌ๋ฆฝํธ] ์คํ์ปจํ ์คํธ (0) | 2023.12.27 |
[์๋ฐ์คํฌ๋ฆฝํธ] ์ค์ฝํ (0) | 2023.12.27 |
[์๋ฐ์คํฌ๋ฆฝํธ] This (0) | 2023.12.27 |